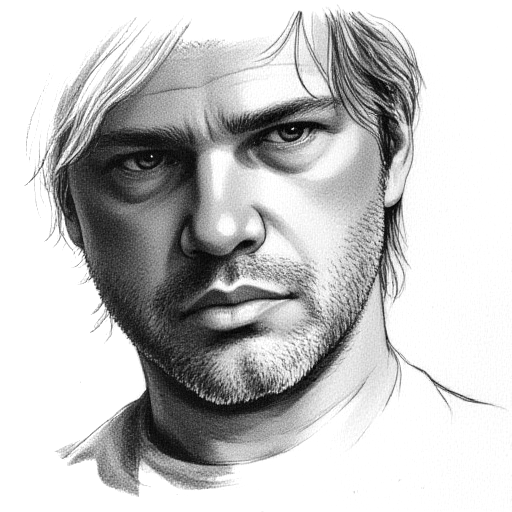
About
I'm an Australian software engineer, married to a librarian from Ohio. We live in Alameda with two children.
I tweet more than I blog. I guess I'm trying to be in the fediverse more now. I'm usually hacking on something or other.
I work for Google on Fuchsia. I'm not looking for a new job. I used to work for start-ups.
The best way to get in touch with me is to email me at ian@mckellar.org.
Posts
Projects
- A Static Site
- How I built this web site.
Old Content
There was some older content that was on my WordPress blog that I've moved over here without comments, probably poorly formatted.